We have a Raspberry Pi driven digital calendar in the kitchen. I’m currently using crontab to turn the HDMI port off at night, and when we’re at work.
But a much more flexible solution is to control it using MQTT and Home Assistant. That way it can easily be automated to turn on when we’re home, if there is movement in the kitchen, or any number of conditions.
It’s really quite easy to do, here’s how 👇
Table of contents
The Python script
On the Raspberry Pi; I’m running a tiny Python script with the paho-mqtt library:
import paho.mqtt.client as mqtt
import time
import subprocess
# The callback for when the client receives a CONNACK response from the server.
def on_connect(client, userdata, flags, rc):
print("Connected with result code " + str(rc))
# Subscribing in on_connect() means that if we lose the connection and
# reconnect then subscriptions will be renewed.
client.subscribe("rpi-board/screen/set")
# The callback for when a PUBLISH message is received from the server.
def on_message(client, userdata, msg):
print(msg.topic + " " + str(msg.payload.decode('utf-8')))
subprocess.run(["vcgencmd", "display_power", str(msg.payload.decode('utf-8'))])
client.publish("rpi-board/screen", get_screen_status())
# Return the state of the HDMI port power
def get_screen_status():
status = subprocess.run(["vcgencmd", "display_power"], stdout=subprocess.PIPE, text=True)
status_b = status.stdout.split("=")[1].strip()
return status_b
client = mqtt.Client("rpi-board")
client.on_connect = on_connect
client.on_message = on_message
client.connect("mqtt.lan.uctrl.net")
client.loop_start()
while True:
client.publish("rpi-board/screen", get_screen_status())
time.sleep(60)
It’s very basic; it subscribes to the rpi-board/screen/set
topic, and runs this shell command whenever it receives a command:
vcgencmd display_power 0/1
With 0/1
being the message payload. This turns the HDMI port on, or off.
On every change, and every minute, it publishes a status message with the topic rpi-board/screen
; indicating the current status of the HDMI port.
To make sure it’s running; I’m using supervisor.
Home Assistant switch
On Home Assistant, I’ve added a MQTT switch, with the topics and payloads described above:
switch:
- platform: mqtt
name: "RPi board screen"
state_topic: "rpi-board/screen"
command_topic: "rpi-board/screen/set"
payload_off: 0
payload_on: 1
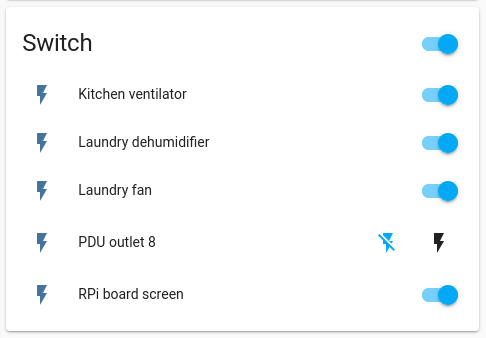
Automating it
Instead of turning the screen off at night, and when we’re at work — I’m planning to use a Aqara motion sensor to turn it on when there is movement in the kitchen. Enabling it when we actually need it, and keeping it off the rest of the time, not relying on a schedule.
I’m just waiting for a Zigbee CC2652P USB stick — so I can get Zigbee2MQTT up and running 🙂
Last commit 2023-12-25, with message: replace emoji slight_smile/slightly_smiling_face